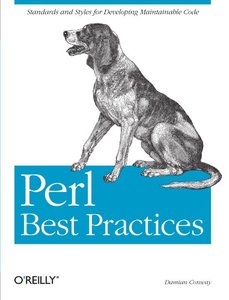
Perl Best Practices (Paperback)
內容描述
Description:
Many programmers code by instinct, relying on
convenient habits or a "style" they picked up early on. They aren't conscious
of all the choices they make, like how they format their source, the names
they use for variables, or the kinds of loops they use. They're focused
entirely on problems they're solving, solutions they're creating, and
algorithms they're implementing. So they write code in the way that seems
natural, that happens intuitively, and that feels good.
But if you're serious about your profession,
intuition isn't enough. Perl Best Practices author Damian Conway
explains that rules, conventions, standards, and practices not only help
programmers communicate and coordinate with one another, they also provide a
reliable framework for thinking about problems, and a common language for
expressing solutions. This is especially critical in Perl, because the
language is designed to offer many ways to accomplish the same task, and
consequently it supports many incompatible dialects.
With a good dose of Aussie humor, Dr. Conway
(familiar to many in the Perl community) offers 256 guidelines on the art of
coding to help you write better Perl code--in fact, the best Perl code you
possibly can. The guidelines cover code layout, naming conventions,
choice of data and control structures, program decomposition, interface design
and implementation, modularity, object orientation, error handling, testing,
and debugging.
They're designed to work together to produce code
that is clear, robust, efficient, maintainable, and concise, but Dr. Conway
doesn't pretend that this is the one true universal and unequivocal set of
best practices. Instead, Perl Best Practices offers coherent and widely
applicable suggestions based on real-world experience of how code is actually
written, rather than on someone's ivory-tower theories on how software ought
to be created.
Most of all, Perl Best Practices offers
guidelines that actually work, and that many developers around the world are
already using. Much like Perl itself, these guidelines are about helping
you to get your job done, without getting in the way.
Table of
Contents:
Preface
- Best Practices
Three Goals This Book
Rehabiting - Code Layout
Bracketing Keywords
Subroutines and Variables Builtins
Keys and Indices Operators
Semicolons Commas
Line Lengths
Indentation Tabs
Blocks Chunking
Elses Vertical Alignment
Breaking Long Lines Non-Terminal
Expressions Breaking by Precedence
Assignments Ternaries
Lists Automated
Layout Naming Conventions
Identifiers Booleans
Reference Variables Arrays and
Hashes Underscores
Capitalization Abbreviations
Ambiguous Abbreviations
Ambiguous Names Utility SubroutinesValues and Expressions
String Delimiters Empty
Strings Single-Character Strings
Escaped Characters Constants
Leading Zeros Long
Numbers Multiline Strings
Here Documents Heredoc Indentation
Heredoc Terminators Heredoc
Quoters Barewords
Fat Commas Thin Commas
Low-Precedence Operators Lists
List Membership- Variables
Lexical Variables Package Variables
Localization
Initialization Punctuation Variables
Localizing Punctuation Variables
Match Variables Dollar-Underscore
Array Indices
Slicing Slice Layout
Slice Factoring - Control Structures
If Blocks Postfix Selectors
Other Postfix Modifiers
Negative Control Statements C-Style Loops
Unnecessary Subscripting
Necessary Subscripting Iterator Variables
Non-Lexical Loop Iterators
List Generation List Selections
List Transformation Complex
Mappings List Processing Side Effects
Multipart Selections Value
Switches Tabular Ternaries
do-while Loops Linear Coding
Distributed Control Redoing
Loop Labels - Documentation
Types of Documentation Boilerplates
Extended Boilerplates
Location Contiguity
Position Technical Documentation
Comments Algorithmic
Documentation Elucidating Documentation
Defensive Documentation
Indicative Documentation Discursive
Documentation Proofreading Built-in Functions
Sorting Reversing Lists
Reversing Scalars Fixed-Width
Data Separated Data
Variable-Width Data String Evaluations
Automating Sorts
Substrings Hash Values
Globbing Sleeping
Mapping and Grepping UtilitiesSubroutines
Call Syntax Homonyms
Argument Lists Named Arguments
Missing Arguments Default
Argument Values Scalar Return Values
Contextual Return Values
Multi-Contextual Return Values Prototypes
Implicit Returns
Returning FailureI/O
Filehandles Indirect Filehandles
Localizing Filehandles
Opening Cleanly Error Checking
Cleanup Input Loops
Line-Based Input Simple
Slurping Power Slurping
Standard Input Printing to Filehandles
Simple Prompting
Interactivity Power Prompting
Progress Indicators Automatic
Progress Indicators AutoflushingReferences
Dereferencing Braced References
Symbolic References Cyclic
ReferencesRegular Expressions
Extended Formatting Line
Boundaries String Boundaries
End of String Matching Anything
Lazy Flags Brace
Delimiters Other Delimiters
Metacharacters Named Characters
Properties
Whitespace Unconstrained Repetitions
Capturing Parentheses
Captured Values Capture Variables
Piecewise Matching Tabular
Regexes Constructing Regexes
Canned Regexes Alternations
Factoring Alternations
Backtracking String ComparisonsError Handling
Exceptions Builtin Failures
Contextual Failure Systemic
Failure Recoverable Failure
Reporting Failure Error Messages
Documenting Errors
OO Exceptions Volatile Error Messages
Exception Hierarchies
Processing Exceptions Exception Classes
Unpacking ExceptionsCommand-Line Processing
Command-Line Structure
Command-Line Conventions Meta-options
In-situ Arguments
Command-Line Processing Interface
Consistency Interapplication ConsistencyObjects
Using OO Criteria
Pseudohashes Restricted Hashes
Encapsulation Constructors
Cloning Destructors
Methods Accessors
Lvalue Accessors
Indirect Objects Class Interfaces
Operator Overloading
Coercions- Class Hierarchies
Inheritance Objects
Blessing Objects Constructor
Arguments Base Class Initialization
Construction and Destruction
Automating Class Hierarchies Attribute
Demolition Attribute Building
Coercions Cumulative Methods
Autoloading - Modules
Interfaces Refactoring
Version Numbers Version Requirements
Exporting
Declarative Exporting Interface Variables
Creating Modules The
Standard Library CPAN - Testing and Debugging
Test Cases Modular Testing
Test Suites Failure
What to Test
Debugging and Testing Strictures
Warnings Correctness
Overriding Strictures The
Debugger Manual Debugging
Semi-Automatic Debugging - Miscellanea
Revision Control Other Languages
Configuration Files
Formats Ties
Cleverness Encapsulated Cleverness
Benchmarking Memory
Caching Memoization
Caching for Optimization
Profiling Enbugging
A. Essential Perl Best
Practices
B. Perl Best
Practices
C. Editor
Configurations
D. Recommended Modules and
Utilities
E. Bibliography
Index